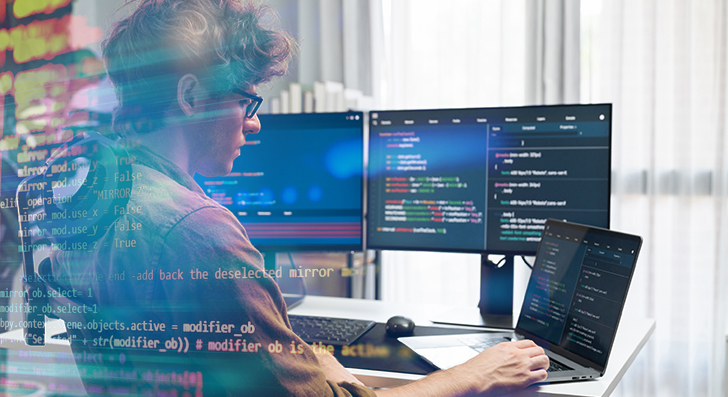
Scalability implies your software can cope with expansion—a lot more customers, extra information, and much more traffic—without breaking. For a developer, creating with scalability in mind will save time and tension afterwards. Right here’s a transparent and functional manual to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element of your prepare from the beginning. A lot of applications fall short when they increase fast due to the fact the original layout can’t handle the extra load. For a developer, you have to Assume early about how your process will behave stressed.
Start out by building your architecture for being versatile. Steer clear of monolithic codebases wherever every thing is tightly linked. Instead, use modular layout or microservices. These styles break your app into scaled-down, unbiased components. Just about every module or service can scale on its own without the need of affecting The full procedure.
Also, take into consideration your database from working day a person. Will it require to manage one million consumers or merely a hundred? Choose the appropriate sort—relational or NoSQL—based upon how your data will increase. System for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital point is to stop hardcoding assumptions. Don’t publish code that only is effective under latest conditions. Think of what would happen Should your consumer foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that aid scaling, like information queues or party-pushed devices. These assist your app deal with more requests without having acquiring overloaded.
Whenever you Develop with scalability in mind, you are not just planning for achievement—you might be cutting down foreseeable future complications. A properly-planned method is easier to take care of, adapt, and increase. It’s far better to get ready early than to rebuild later on.
Use the correct Database
Deciding on the suitable database is really a key Element of making scalable programs. Not all databases are created the identical, and using the Completely wrong one can slow you down or simply bring about failures as your app grows.
Start by knowledge your facts. Could it be highly structured, like rows in a very table? If Certainly, a relational databases like PostgreSQL or MySQL is a good in good shape. These are typically robust with interactions, transactions, and regularity. Additionally they aid scaling tactics like study replicas, indexing, and partitioning to handle additional site visitors and details.
When your data is much more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured details and may scale horizontally much more quickly.
Also, think about your read through and write designs. Are you presently performing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're dealing with a significant write load? Investigate databases which can deal with large produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for momentary data streams).
It’s also sensible to Imagine forward. You might not will need advanced scaling attributes now, but selecting a database that supports them signifies you won’t want to change afterwards.
Use indexing to hurry up queries. Steer clear of needless joins. Normalize or denormalize your facts based upon your access patterns. And often check databases performance as you grow.
In brief, the appropriate databases relies on your app’s structure, speed demands, And exactly how you count on it to expand. Get time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Speedy code is essential to scalability. As your application grows, each individual compact hold off adds up. Inadequately composed code or unoptimized queries can slow down efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Get started by producing clear, straightforward code. Stay away from repeating logic and remove nearly anything unneeded. Don’t choose the most complex Option if an easy 1 is effective. Keep the features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—locations where by your code normally takes as well very long to run or makes use of too much memory.
Upcoming, examine your databases queries. These typically gradual factors down more than the code by itself. Make certain Each individual query only asks for the info you really need. Keep away from SELECT *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout substantial tables.
If you observe a similar info staying requested over and over, use caching. Retail outlet the results briefly working with tools like Redis or Memcached which means you don’t should repeat high-priced functions.
Also, batch your databases operations whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more successful.
Make sure to test with big datasets. Code and queries that do the job good with one hundred information may possibly crash every time they have to take care of 1 million.
In short, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These steps assist your application stay easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more people plus more targeted visitors. If everything goes through a single server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. As opposed to a single server performing all of the work, the load balancer routes users to distinctive servers based upon availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other individuals. Resources like Nginx, HAProxy, or cloud-primarily based options from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it might be reused speedily. When consumers ask for the exact same details again—like an item webpage or a profile—you don’t need to fetch it within the database every time. You may serve it from the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapid access.
two. Client-facet caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and tends to make your application more productive.
Use caching for things which don’t modify normally. And often make certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app take care of extra consumers, keep fast, and Recuperate from challenges. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To build scalable applications, you may need instruments that permit your app develop simply. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A lot smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t really have to buy hardware or guess long term capability. When site visitors will increase, you may insert additional means with just a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app in lieu of running infrastructure.
Containers are A different critical Software. A container offers your application and every little thing it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your application employs numerous containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into companies. You are able to update or scale sections independently, which can be great for performance and dependability.
In short, applying cloud and container equipment means you may scale quick, deploy quickly, and Recuperate promptly when difficulties materialize. If you'd like your application to develop devoid of limits, start out using these equipment early. They help you save time, lower danger, and allow you to continue to be focused on constructing, not correcting.
Check Anything
In the event you don’t keep an eye on your software, you won’t know when issues go Erroneous. Checking assists you see how your application is accomplishing, spot concerns early, and make superior choices as your app grows. It’s a critical Element of developing scalable techniques.
Start out by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—watch your application much too. Keep an eye on how long it requires for end users to load web pages, how frequently glitches transpire, and wherever they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going click here on within your code.
Build alerts for significant challenges. One example is, In the event your reaction time goes earlier mentioned a Restrict or even a support goes down, you ought to get notified right away. This assists you repair concerns quickly, frequently prior to customers even notice.
Checking is likewise valuable once you make modifications. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it triggers real destruction.
As your app grows, visitors and details enhance. Without having checking, you’ll miss indications of difficulty until it’s far too late. But with the correct applications in position, you stay on top of things.
In brief, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for significant organizations. Even compact apps require a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you are able to Make apps that expand effortlessly with out breaking stressed. Get started tiny, Imagine large, and Create good.